TurboImage
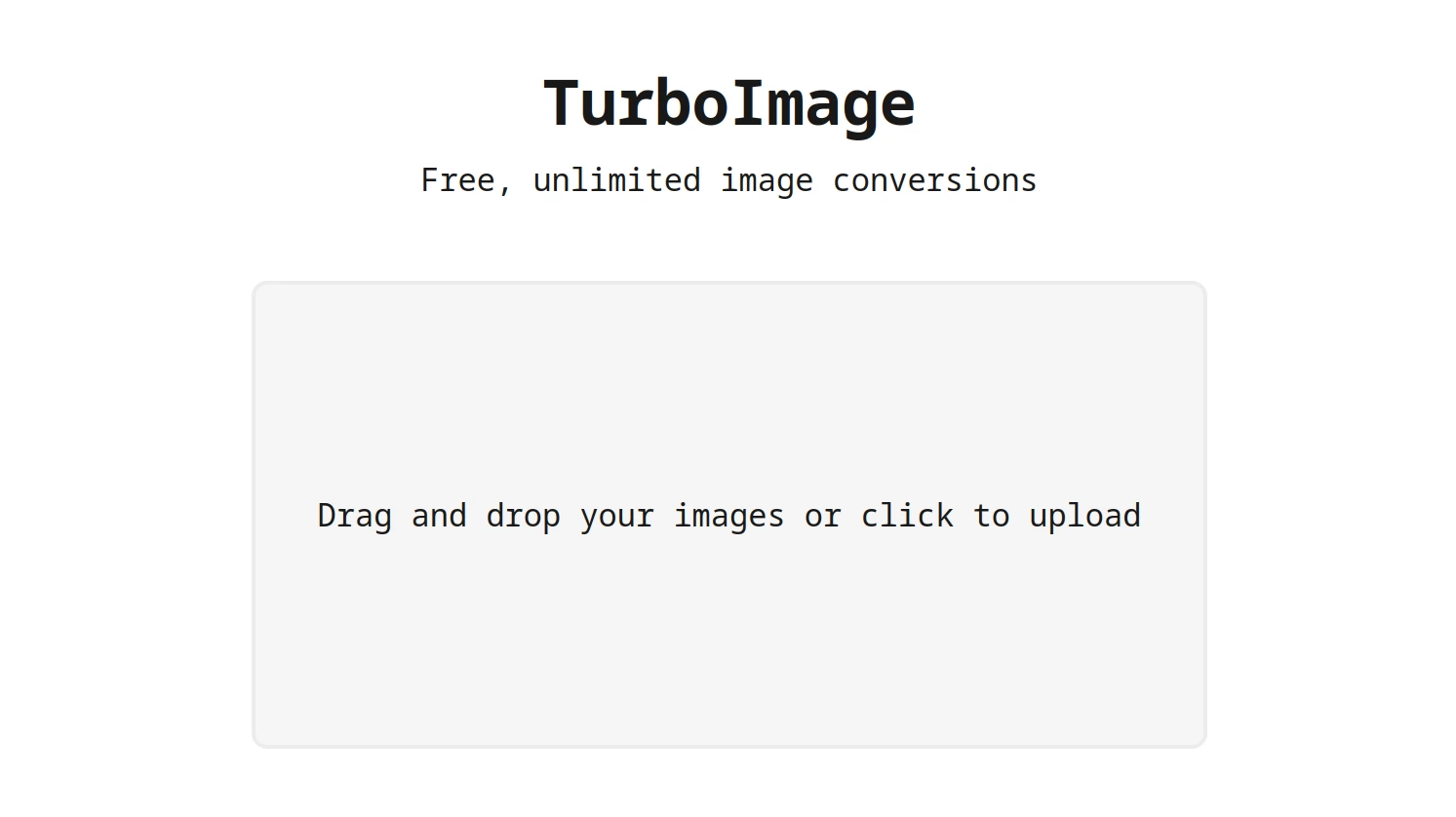
Case
Build an image converter which runs completely in the browser.
Quick links
An image converter is a tool that allows you to change an image’s file format. For example: image.jpg
→ image.png
. TurboImage is a side project I started on my own after facing frustrations with existing image converters. Often, there are limits to how many images you can convert at once, long loading times, or limits on how many images you can convert per hour.
The reason for these limits is the cost of converting images on the server. Image conversion must be done on the server to support the widest range of formats, since creating images directly in the browser only supports a few common formats.
However, when I need to convert images, I usually want to convert to one of these common formats. This means that in theory, I could let my browser do all the work of converting the image without having to be reliant on a (third party) server. To build this converter, we just need an interface that lets us set conversion options like file format and image compression.
Tech stack
Another reason I started this project is because the Svelte 5 beta had just released. Svelte is a framework I like to work with on personal projects for its simplicity and quick setup. The changes made in this newest version looked interesting, so I wanted to explore this new version in a technically advanced project. For this project, my entire tech stack consists of Svelte 5 (with SvelteKit) and TypeScript for type checking. Since I want this converter to work entirely on the client, we won’t be using any server components.
Image conversion
We’ll use the HTML Canvas API to convert images. Provided the file we input is supported by the canvas api of the browser we’re using, we can draw the image onto our canvas. Once the image is drawn, we can simply convert it to any of the supported output formats. Note that not all input formats are also supported as an output format. Here’s a simplified code snippet on how this works:
const convertImage = async (input: UploadedFile) => {
const image = new Image();
image.src = URL.createObjectURL(input.file);
await new Promise<void>((resolve, reject) => {
image.onload = () => resolve();
image.onerror = reject
});
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
ctx.drawImage(image, 0, 0);
const blob = await new Promise<Blob | null>((resolve) =>
canvas.toBlob((blob) => resolve(blob), input.outputMime),
);
};
Browser support
Besides the limited support for image formats, another thing this project relies on is certain FileSystem APIs. We use these APIs to get access to the images that the user wants to upload but also to write the converted images to the user’s disk. One of these APIs we rely on is createWritable. This API is interesting because it allows us to stream a file directly to the user’s disk. The alternative to streaming a file onto the user’s disk is loading the entire file into memory and downloading it all at once. This can cause the entire browser to freeze when working with large files.
Fortunately, an excellent ponyfill exists for this functionality. This ponyfill falls back to using a service worker if the browser does not support the functionality natively. This service worker is able to stream the file to our client somewhat like a server would be able to. All credit for this ponyfill goes to the module author.
Zip streaming
Now that we have the permissions part out of the way, we still have to get to the logic of actually writing a file to the user’s disk. We do this in two different ways:
- Downloading a single image (easy)
- Downloading a single zip with multiple images (hard)
Since downloading a single image takes no more than converting the image and simply downloading it, I want to focus on the creation of a zip file containing any amount of images. To do this, we use a generator, which allows us to store and write data in chunks, rather than all at once. Using this generator we convert our images in batches of a few megabytes and stream them to a zip file. The creation of this zip file is handled by fflate, a lightweight and efficient (de)compression package. Here’s a simplified example of how this all works together:
const convertImages = async () => {
for (const batch of batches) {
const promises = batch.map((input) => convertImage(input));
const results = await Promise.all(promises);
for (const result of results) {
yield result;
}
}
};
const handleZipDownload = async (files: UploadedFile[]) => {
const zip = new Zip();
const rs = new ReadableStream({
start(controller) {
zip.ondata = (err, dat, final) => {
if (err) {
controller.error(err);
} else {
controller.enqueue(dat);
if (final) controller.close();
}
};
},
});
const fileHandle = await showSaveFilePicker({ suggestedName: 'images.zip' });
const writableStream = await fileHandle.createWritable();
rs.pipeTo(writableStream);
for await (const convertedImage of imageGenerator) {
const file = new ZipPassThrough(convertedImage.filename);
zip.add(file);
const reader = convertedImage.blob.stream().getReader();
while (true) {
const { value, done } = await reader.read();
if (done) {
file.push(new Uint8Array(0), true);
break;
}
file.push(value);
}
reader.cancel();
}
zip.end();
};
The result
What began as a way to learn a new version of Svelte and build a quick and dirty image converter prototype, ended as an optimized, customizable web application with way more features than I will realistically ever use. If you want to give Turbo Image a try, you can view the project here.