Chaos cards
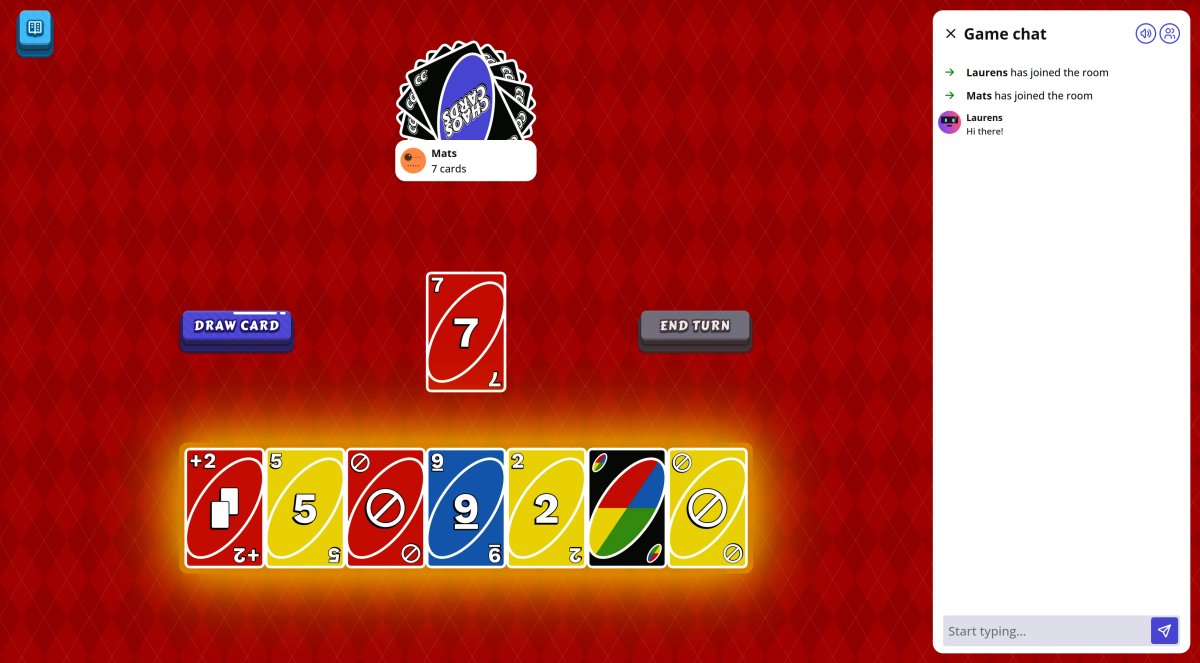
Case
Build an online multiplayer game that seeing people and/or visually impaired people using a screenreader can play together
Quick links
Chaos Cards is an online game I created for my bachelor’s graduation project. It has the same rules as popular card game: Uno. The game can be played in realtime by people anywhere over the internet. The added twist is that this game is also entirely playable by (blind) people using a screenreader.
What is a screenreader?
A screenreader is a piece of software that reads out the contents of a screen to a user. This is useful for people with visual impairments, which was my target audience for this project. The screenreader reads out the text on the screen and can also read out the contents of buttons, links and other interactive elements. By using their keyboard, a user can navigate through the website and interact with it.
Tech stack
For this project the tech stack I decided on consists of: Next.js frontend, Express server, Typescript, and Socket.io for communication between the server and client. The reason I chose this tech stack is I had the most experience with these tools working on large scale projects, and we had a relatively short time to build a working prototype.
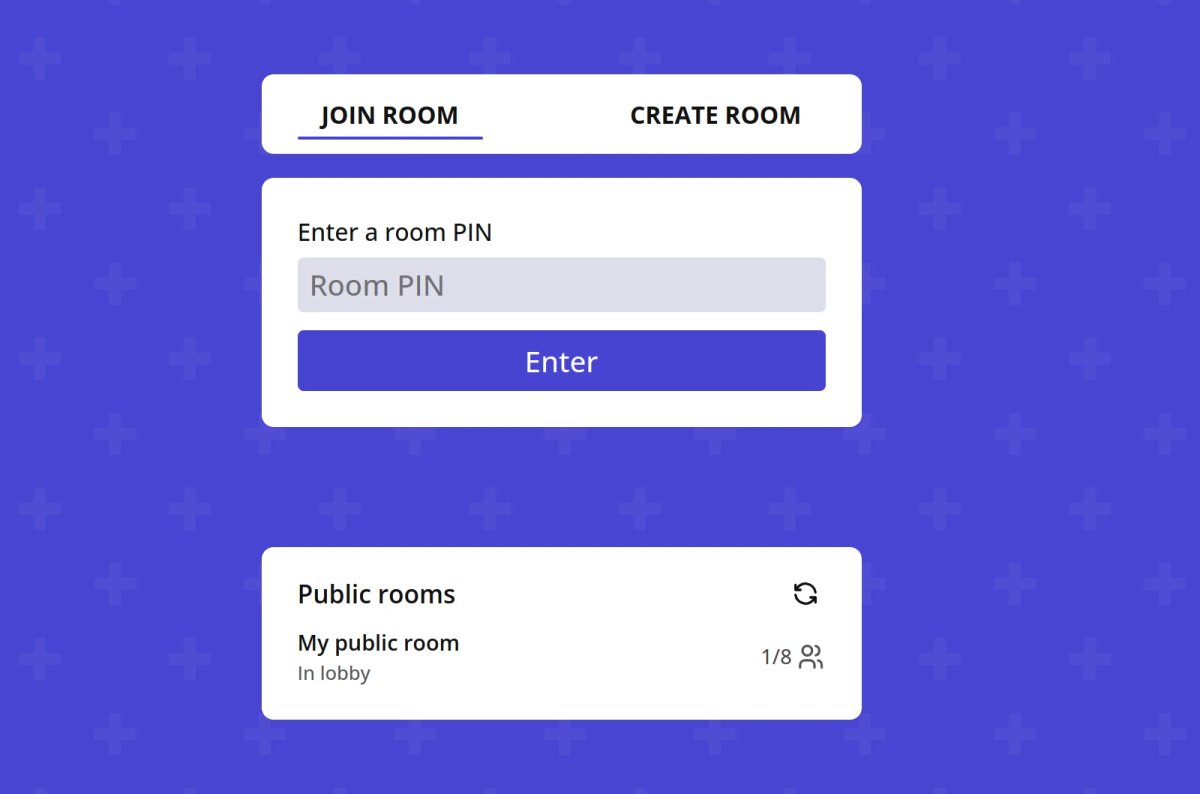
How does it work
To be able to play a video game in realtime, we have to make sure the client can communicate with the server quickly. To achieve this, we use Socket.io, a library which uses Websockets to create an open connection between the client and the server. Using this connection, the client and server are able to communicate much quicker than we would be able to using normal HTTP requests.
Accessibility
A large portion of the website relies on simple, native, html elements and attributes. These elements and attributes are implemented according to the WCAG (Web Content Accessibility Guidelines) spec. These guidelines describe best practices and rules to follow when building a website to make it as accessible as possible.
The more complicated part of this project (the interaction) builds upon this spec with some creative logic to make the interaction more or less the same for people using a screenreader and people not using a screenreader.
Implementation
The reason why the interactive aspect of this project is possible is because of the aria-live attribute. This attribute tells the browser to make the screenreader announce something, even when the user does not manually trigger this interaction. This attribute can be used to make screenreaders read notifications for example, which is similar to what we’re doing when we want to make the screenreader read when someone has played a card. I use the following React component to store the complete game history, and make a screenreader read the most recent history item.
const GameHistory = ({ entries, urgency = 'assertive' }: GameHistoryProps) => (
<section className="visuallyHidden">
<ol aria-label="game history">
{entries.map(({ key, entry }) => (
<li key={key}>{entry}</li>
))}
<li>The game started</li>
</ol>
<p aria-live={urgency}>{entries[0]?.entry}</p>
</section>
);
All we need to pass to this component are clear descriptions of what “actions” can happen throughout a game. For example: someone playing a certain card with a certain number or someone ending their turn. Since we know all possible actions in advance, we can write these messages where the code handles the actions.
Validation
Throughout the project, I tested the usability of the prototype with both seeing- and visually impaired people, since the goal of the project was to allow seeing and visually impaired people to play together. For testing with visually impaired people, I reached out to Bartiméus, an interest group for the visually impaired. Here, I got in contact with Mike, who helped me test and improve my prototype several times. The testing with seeing people was done by my teachers, and fellow students.
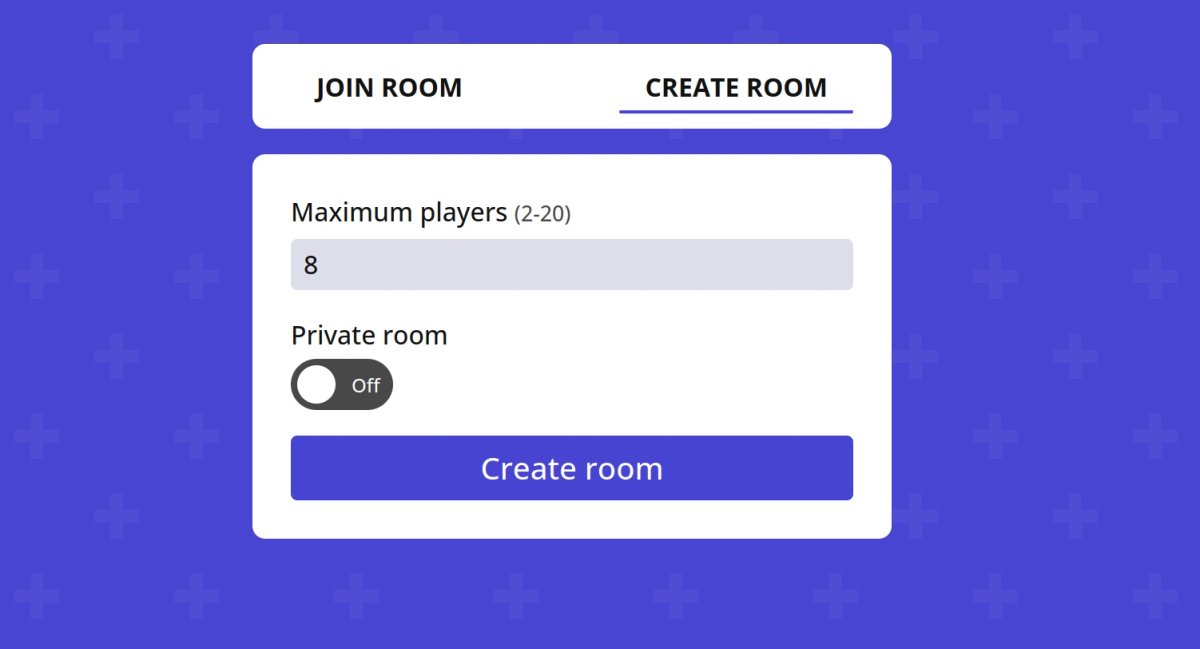
Result
The result of this project is a fully working prototype of a game that can be played by both seeing and visually impaired people. Chaos cards is fully playable with- or without a screenreader, and can be played in realtime with people anywhere in the world. There is no online demo for this project because of the involved costs of running a server and the fact that this is a prototype not optimized for a production environment. For a full walkthrough of the application, you can view the video below. Note that I’m using a screenreader in this demo, but any of the interactions would work the same using a mouse or touchscreen.
If you wish, you can also run the project locally with the code from my Github repo.